如何在 5 维 numpy 矩阵中旋转时间序列?
How do I rotate timeseries in a 5-d numpy matrix?
我正在尝试旋转保存在 numpy nd-array 中的一些地震数据。该数组具有维度(N-receiver、M-sources、3-source_channels、3 个接收通道、K-time 个通道)。
我知道如何在单个接收器和单个源站上应用于单个时间戳 (t_i) 时设置旋转。实际的 Z、R、T、N、E 符号对于一般问题并不重要,只要知道变换是这样定义的即可:
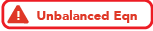&space;&&space;ZR(t_i)&&space;ZT(t_i)&space;%5C%5C&space;RZ(t_i)&space;&&space;RR(t_i)&space;&&space;RT(t_i)&space;%5C%5C&space;TZ(t_i)&space;&&space;TR(t_i))&space;&&space;TT(t_i)&space;%5Cend%7Bvmatrix%7D&space;=%5Cbegin%7Bvmatrix%7D&space;1&space;&&space;0&space;&&space;0&space;%5C%5C&space;0&space;&&space;cos(%5Calpha)&space;&&space;sin(%5Calpha)&space;%5C%5C&space;0&space;&&space;-sin(%5Calpha)&space;&&space;cos(%5Calpha)&space;%5Cend%7Bvmatrix%7D&space;%5Cbegin%7Bvmatrix%7D&space;ZZ(t_i)&space;&&space;ZN(t_i)&space;&&space;ZE(t_i)&space;%5C%5C&space;NZ(t_i)&space;&&space;NN(t_i)&space;&&space;NE(t_i)&space;%5C%5C&space;EZ(t_i)&space;&&space;EN(t_i)&space;&&space;EE(t_i)&space;%5Cend%7Bvmatrix%7D&space;%5Cbegin%7Bvmatrix%7D&space;1&space;&&space;0&space;&&space;0&space;%5C%5C&space;0&space;&&space;-cos(%5Cbeta)&space;&&space;-sin(%5Cbeta)&space;%5C%5C&space;0&space;&&space;sin(%5Cbeta)&space;&&space;-cos(%5Cbeta)&space;%5Cend%7Bvmatrix%7D)
在 python 中,对于单个时间戳,我可能会编写如下代码:
import numpy as np
a = 50.0 # example alpha
b = 130 # example beta
a_rotation = np.asarray([[1,0,0],[0,np.cos(a),np.sin(a)],[0,-np.sin(a),np.cos(a)]])
b_rotation = np.asarray([[1,0,0],[0,-np.cos(b),-np.sin(b)],[0,np.sin(b),-np.cos(b)]])
# pretend the zn's are actual float data
single_timeslice_data = np.asarray([[zz,zn,ze],[nz,nn,ne],[ez,en,ee]])
# operation w numpy matrix rotation
rotated_channels = a_rotation @ single_timeslice_data @ b_rotation
所以我的问题是双重的:
- 如何在所有时间步长上应用这个带有 numpy 的矩阵乘积?
例如:(3 x 3) * (3 x 3 x K) * (3 x 3)
- 当可能有任意数量的其他维度时,如何使用 numpy 进行矩阵乘积?
ex: (3 x 3) * (N x M x 3 x 3 x K) * (3 x 3)
1)
(3 x 3) * (3 x 3 * K) * (3 * 3) = (3 x 3 x K)
np.einsum('ab,bcK,cd->adK', Arr1, Arr2, Arr3)
2)
(3 x 3) * (N x M x 3 x 3 x K) * (3 x 3) = (N x M x 3 x 3 x K)
np.einsum('ab,NMbcK,cd->NMadK', Arr1, Arr2, Arr3)
我正在尝试旋转保存在 numpy nd-array 中的一些地震数据。该数组具有维度(N-receiver、M-sources、3-source_channels、3 个接收通道、K-time 个通道)。
我知道如何在单个接收器和单个源站上应用于单个时间戳 (t_i) 时设置旋转。实际的 Z、R、T、N、E 符号对于一般问题并不重要,只要知道变换是这样定义的即可:
在 python 中,对于单个时间戳,我可能会编写如下代码:
import numpy as np
a = 50.0 # example alpha
b = 130 # example beta
a_rotation = np.asarray([[1,0,0],[0,np.cos(a),np.sin(a)],[0,-np.sin(a),np.cos(a)]])
b_rotation = np.asarray([[1,0,0],[0,-np.cos(b),-np.sin(b)],[0,np.sin(b),-np.cos(b)]])
# pretend the zn's are actual float data
single_timeslice_data = np.asarray([[zz,zn,ze],[nz,nn,ne],[ez,en,ee]])
# operation w numpy matrix rotation
rotated_channels = a_rotation @ single_timeslice_data @ b_rotation
所以我的问题是双重的:
- 如何在所有时间步长上应用这个带有 numpy 的矩阵乘积?
例如:(3 x 3) * (3 x 3 x K) * (3 x 3)
- 当可能有任意数量的其他维度时,如何使用 numpy 进行矩阵乘积?
ex: (3 x 3) * (N x M x 3 x 3 x K) * (3 x 3)
1)
(3 x 3) * (3 x 3 * K) * (3 * 3) = (3 x 3 x K)
np.einsum('ab,bcK,cd->adK', Arr1, Arr2, Arr3)
2)
(3 x 3) * (N x M x 3 x 3 x K) * (3 x 3) = (N x M x 3 x 3 x K)
np.einsum('ab,NMbcK,cd->NMadK', Arr1, Arr2, Arr3)